godot-logger – A Rust Logger for Godot
While building a game with Rust and Godot, I wanted to see log messages in Godot's editor. Because I could not find an existing solution, I went ahead and created godot-logger, a crate that prints to Godot's output console.
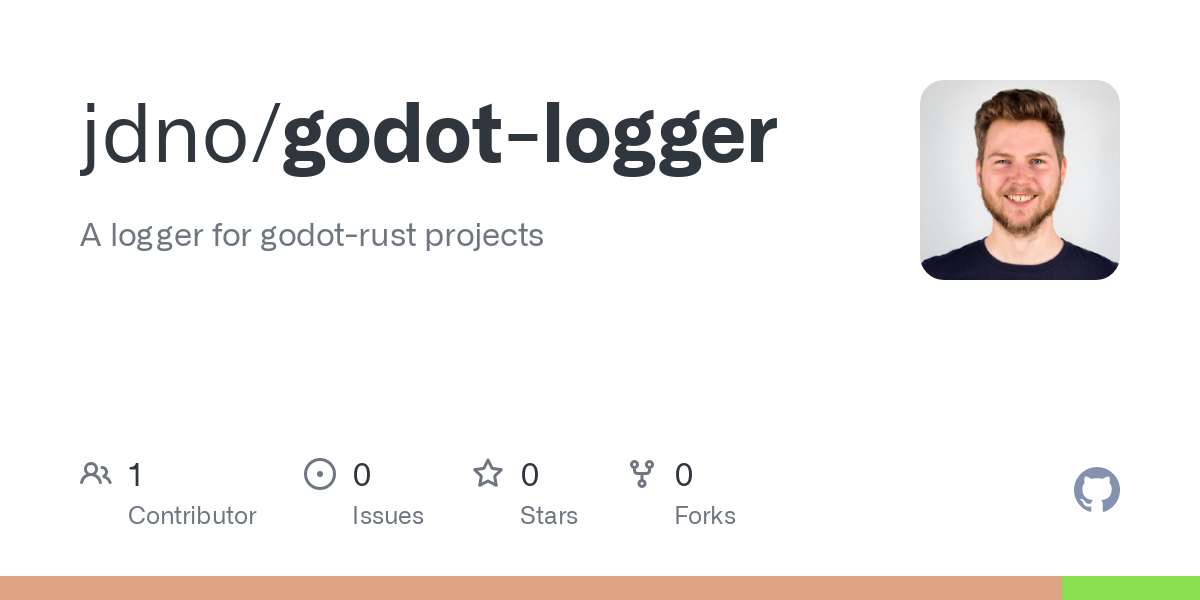
godot-logger is a crate that complements the godot-rust bindings for Godot, and prints logs to Godot's output console using the godot_print!
and godot_warn!
macros. It is available as open source on GitHub.
Introduction
Godot is an open source game engine with a powerful editor, tools to debug and profile the game, and support for many different programming langauges. Building games with Rust is possible thanks to the godot-rust library, which provides bindings to Godot's API as well as a few convenience methods.
One of the debugging tools inside Godot is the output console. It prints information about the execution of the game, as well as log messages that have been placed in the code.
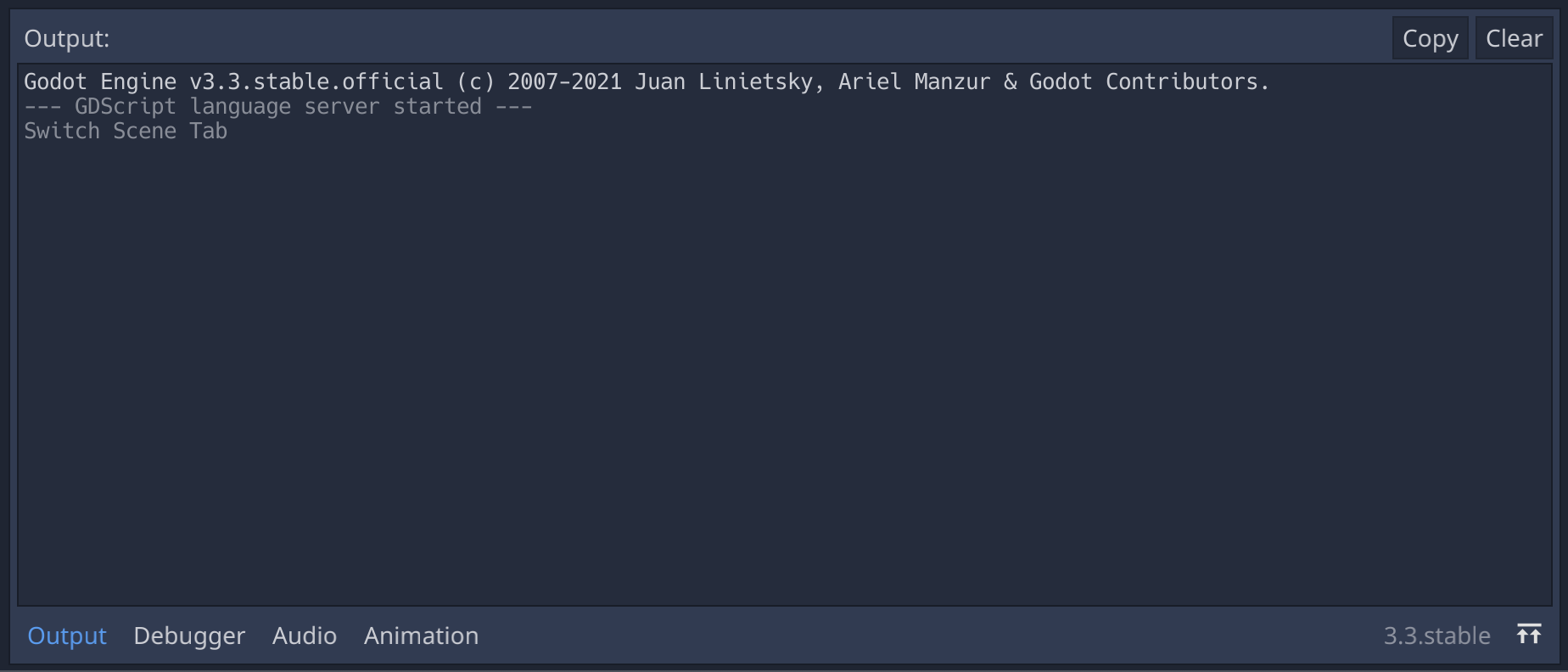
godot-rust provides two macros to write to the output console: godot_print!
and godot_warn!
. These can be called anywhere in the code, and will print the given message to the console.
The problem that I encountered while working on my game was that these macros do not capture logs from third-party Rust libraries. It is very common for libraries in Rust to use the log crate and its logging facade to define log statements in their code. These messages can then be printing by initializing a logger such as env_logger or log4rs. I wanted to see what the libraries in my project were doing, and I wanted to see the logs inside Godot's editor where I was running the game. After searching for an existing library and coming up empty, I decided to implement a logger myself that would print to Godot's output console.
Usage
godot-logger is very similar to popular logging frameworks such as env_logger or log4rs. It integrates with the logging facade of the log crate, and prints log statements to Godot's output console.
The simplest way to use godot-logger is to add it and the log crate as dependencies to your project's Cargo.toml
:
[dependencies]
godot-logger = "1.0"
log = "0.4
Then configure and initialize the logger in the same method that is passed to godot_init!
.
use gdnative::prelude::*;
use godot_logger::GodotLogger;
use log::{Level, LevelFilter};
fn init(handle: InitHandle) {
GodotLogger::builder()
.default_log_level(Level::Info)
.add_filter("godot_logger", LevelFilter::Debug)
.init();
log::debug!("Initialized the logger");
}
godot_init!(init);
The example shows the configuration options that godot-logger provides:
- The default log level of
GodotLogger
isLevel::Warn
, but this can be changed withdefault_log_level
. - Module-specific log levels that take precedence over the default log level can be set by adding filters to the logger. A filter combines a module path and a level filter, which makes it possible to very granularly control what gets logged.
The example above will print the following line to the console:
2021-10-20 20:00:00 DEBUG godot_logger Initialized the logger
Below is a screenshot from my own project, which runs an embedded server built with Bevy and has its code split across a few different crates ( aomos
namespace). As you can see, log
statements in these libraries are picked up and printed inside Godot.
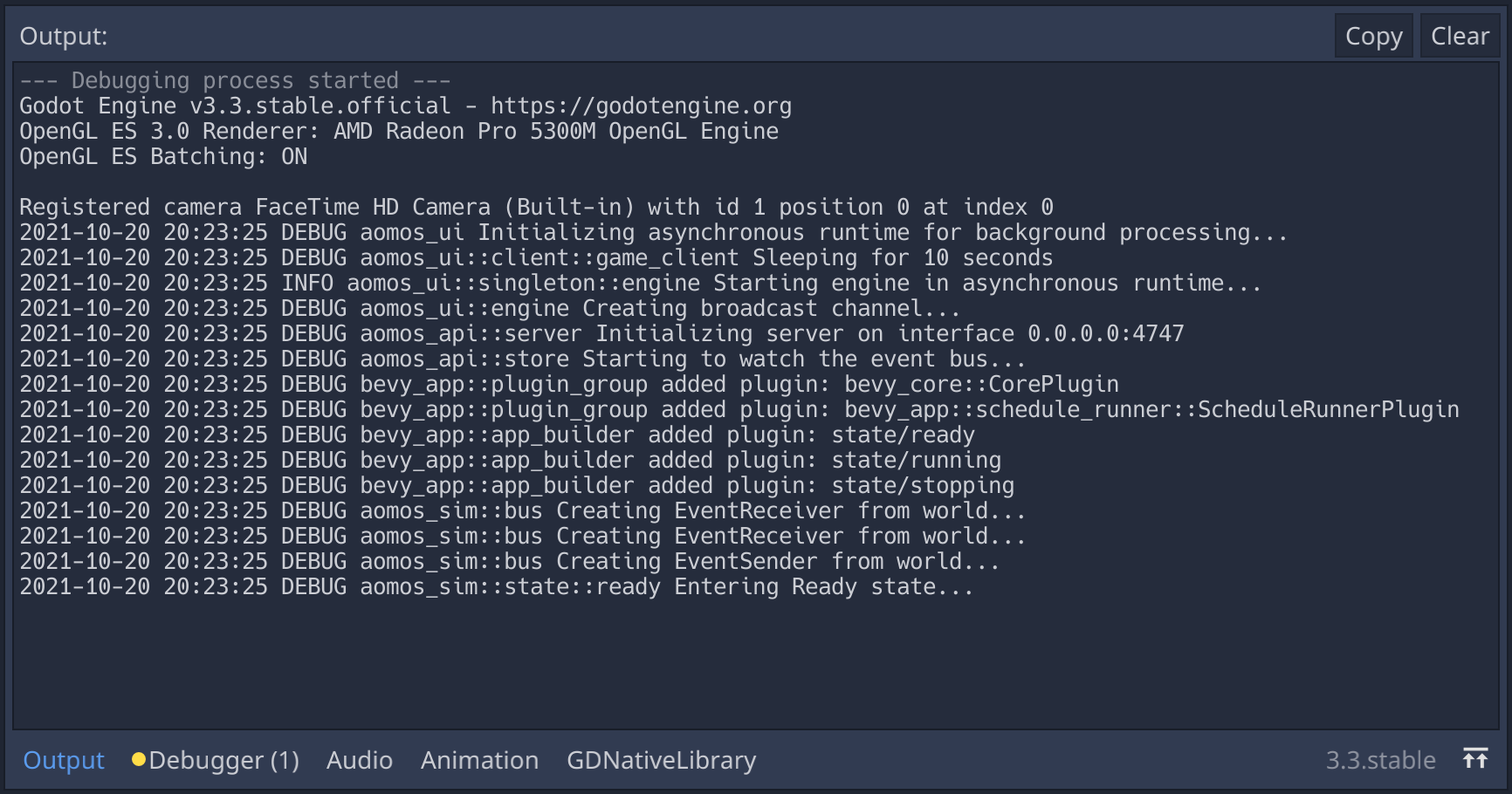
Contributing
godot-logger is published as an open source project on GitHub, and bug reports are always welcome.